JavaScript
- Description
- Curriculum
- Reviews
- Grade
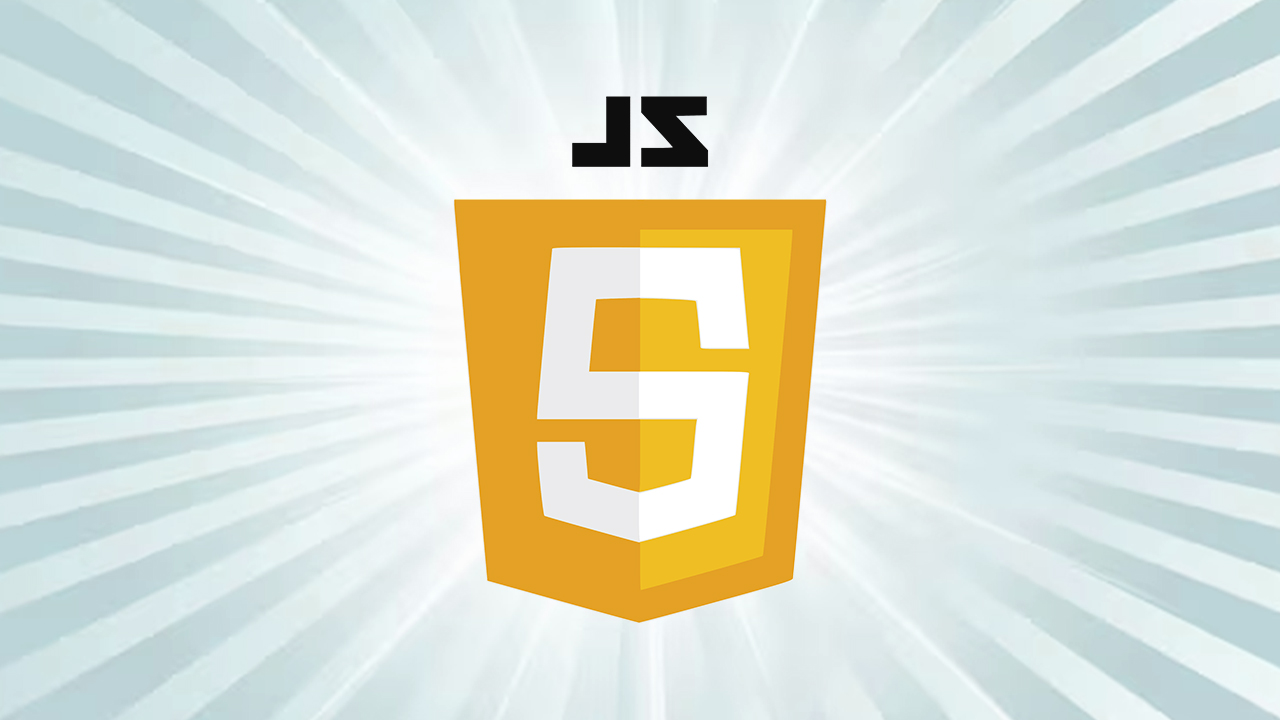
Course Title: JavaScript: A Comprehensive Guide for Beginners
Course Overview:
Welcome to the JavaScript Course, an in-depth program designed to help you understand and master JavaScript, one of the most widely used programming languages for web design and development. Whether you’re a complete beginner or someone looking to enhance your programming skills, this course will provide you with everything you need to build interactive, dynamic, and functional websites using JavaScript.
In this course, you will start with the basics of JavaScript and gradually progress to more advanced concepts, learning how to add functionality, manipulate data, handle events, and interact with the web page and the user. By the end of this course, you’ll be able to write efficient JavaScript code that can create engaging user experiences, as well as set a strong foundation for learning more advanced web technologies.
What You Will Learn:
– Introduction to JavaScript: Understand what JavaScript is, how it works, and its role in web design and development alongside HTML and CSS.
– Basic JavaScript Syntax: Learn how to write basic JavaScript code, including variables, data types, operators, and expressions.
– Control Structures: Master the use of conditionals (if, else, switch) and loops (for, while, do-while) to control the flow of your program.
– Functions: Understand how to define and call functions, pass parameters, and return values to create reusable, modular code.
– DOM Manipulation: Learn how to interact with and modify HTML elements dynamically using JavaScript, allowing you to create interactive web pages.
– Event Handling: Discover how to handle user input and actions (like clicks, key presses, and mouse movements) using JavaScript event listeners.
– Arrays and Objects: Learn how to work with collections of data using arrays and objects, and how to perform operations such as adding, removing, and updating elements.
– Asynchronous JavaScript: Understand how to work with asynchronous code using callbacks, promises, and async/await to manage tasks like fetching data from an API.
– Error Handling: Learn how to handle errors in your code effectively using try, catch, and finally to ensure smooth execution and user experience.
– Debugging and Best Practices: Learn essential debugging techniques, tools, and best practices for writing clean, efficient, and maintainable JavaScript code.
Who Is This Course For?
– Beginners with no prior programming experience who want to learn JavaScript from scratch.
– Aspiring web developers looking to add interactivity and functionality to their websites.
– Front-end developers who want to deepen their knowledge of JavaScript and improve their coding skills.
– Anyone interested in learning how JavaScript powers the web and how it can be used to create dynamic, interactive websites.
– Programmers from other languages looking to transition into web design and development and JavaScript.
Why Take This Course?
– Comprehensive Curriculum: This course provides a complete guide to JavaScript, from basic syntax to advanced concepts, ensuring you have a thorough understanding of the language.
– Hands-On Projects: Put your learning into practice with real-world projects, such as building interactive web pages, games, and applications.
– Interactive Lessons: Engage with rich multimedia lessons, including text-based tutorials, video demonstrations, quizzes, and coding exercises to reinforce key concepts.
– Expert Instructor: Learn from an experienced instructor who explains complex JavaScript concepts in a simple, easy-to-understand manner, with plenty of practical examples.
– Lifetime Access: Enjoy lifetime access to all course materials, so you can learn at your own pace, review lessons, and stay updated with the latest JavaScript developments.
Course Requirements:
– A computer or mobile device with internet access to watch videos and follow along with exercises.
– Basic knowledge of Text editor (Notepad)
– Basic knowledge of Browser (Chrome)
– Basic knowledge of HTML and CSS is helpful, but not required.
– No prior programming experience needed—this course is designed for absolute beginners.
Course Duration:
– Total Duration: Self-paced learning, with flexible timelines to fit your schedule.
Course Format:
– Lessons: In-depth video tutorials, hands-on coding examples, and demonstrations of key concepts.
– Quizzes: Interactive quizzes to test your understanding of the material and reinforce learning.
– Assignments: Real-world coding challenges to practice and apply what you’ve learned.
– Discussion Forum: Participate in a community of learners to ask questions, share projects, and get feedback from both the instructor and fellow students.
Certification:
Upon successful completion of the course, you will receive a Certificate of Completion, which you can showcase on your resume or LinkedIn profile to demonstrate your expertise in JavaScript and web design and development.
-
1Hello World program in JavaScript
-
2Multiple Spaces and Line break in JavaScript
Multiple Spaces and Line break in JavaScript
-
3Comments in JavaScript
Comments in JavaScript
-
4How to use HTML Tags in JavaScript
How to use HTML Tags in JavaScript
-
5How to use HTML Attributes in JavaScript
How to use HTML Attributes in JavaScript
-
6How to create HTML Table Layout in JavaScript
How to create HTML Table Layout in JavaScript
-
7Addition / Concatenation Operator in JavaScript
Addition / Concatenation Operator in JavaScript
-
8document.write() in JavaScript
document.write() in JavaScript
-
9Keywords in JavaScript
Keywords in JavaScript
-
10Identifiers in JavaScript
Identifiers in JavaScript
-
11Variables in JavaScript
Variables in JavaScript
-
12Constants in JavaScript
Constants in JavaScript
-
13Naming Conventions in JavaScript
Naming Conventions in JavaScript
-
14Data Types in JavaScript
Data Types in JavaScript
-
15Types of Data Types in JavaScript
Types of Data Types in JavaScript
-
16Type Casting in JavaScript
Type Casting in JavaScript
-
17parsetInt() Function in JavaScript
parsetInt() Function in JavaScript
-
18parseFloat() Function in JavaScript
parseFloat() Function in JavaScript
-
19eval() Function in JavaScript
eval() Function in JavaScript
-
20Operators in JavaScript
Operators in JavaScript
-
21Arithmetic Operators in JavaScript
Arithmetic Operators in JavaScript
-
22Relational Operators in JavaScript
Relational Operators in JavaScript
-
23Logical Operators in JavaScript
Logical Operators in JavaScript
-
24Conditional Operator in JavaScript
Conditional Operator in JavaScript
-
25Assignment Operators in JavaScript
Assignment Operators in JavaScript
-
26Increment and Decrement Operators in JavaScript Part 1
Increment and Decrement Operators in JavaScript Part 1
-
27Increment and Decrement Operators in JavaScript Part 2
Increment and Decrement Operators in JavaScript Part 2
-
28Binary vs. Decimal Number System
Binary vs. Decimal Number System
-
29Bitwise Operators in JavaScript
Bitwise Operators in JavaScript
-
30Special Operators in JavaScript
Special Operators in JavaScript
-
31JavaScript Operator Precedence and Associativity
JavaScript Operator Precedence and Associativity
-
32Control Statements in JavaScript
Control Statements in JavaScript
-
33if and if else in JavaScript
if and if else in JavaScript
-
34if-else vs Ternary Operator in JavaScript
if-else vs Ternary Operator in JavaScript
-
35if-else-if statement in JavaScript
if-else-if statement in JavaScript
-
36switch case Statement in JavaScript
switch case Statement in JavaScript
-
37How to Debug JavaScript in Chrome
How to Debug JavaScript in Chrome
-
38How to Debug JavaScript in Firefox
How to Debug JavaScript in Firefox
-
39Loops in JavaScript
Loops in JavaScript
-
40for loop in JavaScript
for loop in JavaScript
-
41Forward for loop in JavaScript
Forward for loop in JavaScript
-
42Reverse for loop in JavaScript
Reverse for loop in JavaScript
-
43while loop in JavaScript
while loop in JavaScript
-
44When to use While loop in JavaScript
When to use While loop in JavaScript
-
45Find Sum of Digits using While loop
Find Sum of Digits using While loop
-
46do while loop in JavaScript
do while loop in JavaScript
-
47for in loop in JavaScript
for in loop in JavaScript
-
48break Statement in JavaScript
break Statement in JavaScript
-
49Linear Search algorithm in JavaScript
Linear Search algorithm in JavaScript
-
50continue Statement in JavaScript
continue Statement in JavaScript
-
51continue inside JavaScript while and do while loop
continue inside JavaScript while and do while loop
-
52JavaScript Nested loops with Same max value
JavaScript Nested loops with Same max value
-
53JavaScript Nested loops with Different max value
JavaScript Nested loops with Different max value
-
54Types of Loops in JavaScript
Types of Loops in JavaScript
-
55Print Square Pattern in JavaScript
Print Square Pattern in JavaScript
-
56Print Pyramid Pattern in JavaScript
Print Pyramid Pattern in JavaScript
-
57Functions in JavaScript
Functions in JavaScript
-
58Types of Functions in JavaScript
Types of Functions in JavaScript
-
59JavaScript Function Expression
JavaScript Function Expression
-
60Function Declaration vs Function Expression
Function Declaration vs Function Expression
-
61JavaScript Function Constructor
JavaScript Function Constructor
-
62JavaScript Immediately Invoked Function Expression (IIFE)
JavaScript Immediately Invoked Function Expression (IIFE)
-
63JavaScript Variable Scope
JavaScript Variable Scope
-
64JavaScript Functions and the Call Stack
JavaScript Functions and the Call Stack
-
65How to call function inside another function
How to call function inside another function
-
66Find Factorial using JavaScript for loop
Find Factorial using JavaScript for loop
-
67Find Factorial using JavaScript Recursive function
Find Factorial using JavaScript Recursive function
-
68What is an Object in Programming
What is an Object in Programming
-
69JavaScript Objects Introduction
JavaScript Objects Introduction
-
70Types of Objects in JavaScript
Types of Objects in JavaScript
-
71How to create Objects in JavaScript
How to create Objects in JavaScript
-
72JavaScript Object Constructor Notation
JavaScript Object Constructor Notation
-
73How to add and delete Object properties in JavaScript
How to add and delete Object properties in JavaScript
-
74How to set and get Object Properties in JavaScript
How to set and get Object Properties in JavaScript
-
75Object Literal vs. Object Constructor in JavaScript
Object Literal vs. Object Constructor in JavaScript
-
76Advantages of JavaScript Object Literal notation
Advantages of JavaScript Object Literal notation
-
77Constructor Function in JavaScript
Constructor Function in JavaScript
-
78How to create Objects using JS Object Constructor
How to create Objects using JS Object Constructor
-
79this Keyword in JavaScript
this Keyword in JavaScript
-
80__proto__ vs prototype in JavaScript
__proto__ vs prototype in JavaScript
-
81Math Object in JavaScript
Math Object in JavaScript
-
82Date Object in JavaScript
Date Object in JavaScript
-
83How to create Date Object in JavaScript
How to create Date Object in JavaScript
-
84How to display Date and Time in JavaScript
How to display Date and Time in JavaScript
-
85JavaScript Date Functions
JavaScript Date Functions
-
86JavaScript String Object
JavaScript String Object
-
87JavaScript String Functions
JavaScript String Functions
-
88JavaScript Regular Expressions Part1
JavaScript Regular Expressions Part1
-
89JavaScript Regular Expressions Part2
JavaScript Regular Expressions Part2
-
90JavaScript Regular Expressions Part3
JavaScript Regular Expressions Part3
-
91JavaScript Regular Expressions Part4
JavaScript Regular Expressions Part4
-
92JavaScript Regular Expressions Part5
JavaScript Regular Expressions Part5
-
93JavaScript Regular Expressions Part6
JavaScript Regular Expressions Part6
-
94Phone Number Validation in JavaScript
Phone Number Validation in JavaScript
-
95Email Validation in JavaScript
Email Validation in JavaScript
-
96Arrays in JavaScript
Arrays in JavaScript
-
97Traversing Arrays in JavaScript
Traversing Arrays in JavaScript
-
98JavaScript Array Methods Part1
JavaScript Array Methods Part1
-
99JavaScript Array Methods Part2
JavaScript Array Methods Part2
-
100JavaScript Array Methods Part3
JavaScript Array Methods Part3
-
101JavaScript Array Methods Part4
JavaScript Array Methods Part4
-
102JavaScript Array Methods Part5
JavaScript Array Methods Part5
-
103JavaScript getElementById() method
JavaScript getElementById() method
-
104JavaScript getElementsByClassName()
JavaScript getElementsByClassName()
-
105JavaScript getElementsByTagName()
JavaScript getElementsByTagName()
-
106How to handle JavaScript Events ?
How to handle JavaScript Events ?
-
107How to Add Events to an HTML element
How to Add Events to an HTML element
-
108How to Get current HTML Element in JavaScript
How to Get current HTML Element in JavaScript
-
109JavaScript Events with return value
JavaScript Events with return value
-
110JavaScript setTimeout() function
JavaScript setTimeout() function
-
111JavaScript setInterval() Function
JavaScript setInterval() Function
-
1123 Ways to Add JavaScript to HTML
-
113Addition of Two Numbers App in JavaScript
-
114Area Of Circle Calculator App in JavaScript
-
115Odd or Even Number App in JavaScript
-
116Multiplication Table App in JavaScript
-
117Guess the Number Game in JavaScript
-
118Awesome Calculator App in JavaScript
-
119Make HTML element follow Mouse Cursor in JavaScript
-
120Move HTML element with Mouse in JavaScript
-
121Form Validation in JavaScript Part 1
-
122Form Validation in JavaScript Part 2
-
123Form Validation in JavaScript Part 3
-
124Form Validation in JavaScript Part 4
-
125Form Validation in JavaScript Part 5
-
126Form Validation in JavaScript Part 6
-
127Form Validation in JavaScript Part 7
-
128Automatic Image Slideshow in JavaScript Part 1
-
129Automatic Image Slideshow in JavaScript Part2
-
130Automatic Image Slideshow in JavaScript Part 3
-
131Automatic Image Slideshow in JavaScript Part 4
-
132Automatic Image Slideshow in JavaScript Part 5
-
133Automatic Image Slideshow in JavaScript Part 6
-
134Automatic Image Slideshow in JavaScript Part 7
-
135Automatic Image Slideshow in JavaScript Part 8
-
136Automatic Image Slideshow in JavaScript Part 9
-
137Interactive Image Slideshow in JavaScript Part 1
-
138Interactive Image Slideshow in JavaScript Part 2
-
139Interactive Image Slideshow in JavaScript Part 3
-
140Interactive Image Slideshow in JavaScript Part 4
-
141Interactive Image Slideshow in JavaScript Part 5
-
142Interactive Image Slideshow in JavaScript Part 6
-
143Interactive Image Slideshow in JavaScript Part 7
-
144Interactive Image Slideshow in JavaScript Part 8
-
145Interactive Image Slideshow in JavaScript Part 9
-
146Interactive Image Slideshow in JavaScript Part 10
-
147JavaScript QuizTest your knowledge of JavaScript with this comprehensive 50-question quiz. You have 60 minutes to complete the quiz, and the questions are presented in a paginated format for an organized experience. To pass, you need to score at least 70%. If you retake the quiz, 5% will be deducted from your total score. After completing the quiz, the correct answers will be displayed to help you learn and improve. Good luck!